Multiples of 3 or 5
Say I give you numbers from 1 to 20, and I ask what numbers are divisible by 3 or 5. The numbers are 3, 5, 6, 9, 10, 12, 15, 18, and 20. Also, I would like the sum of those numbers. The sum is 3 + 5 + 6 + 9 + 10 + 12 + 15 + 18 + 20 = 98. This problem is not too difficult to solve, and I will do it using JavaScript and Ruby.
I will solve this using JavaScript first. The first thing we want to do is introduce an array that we want to hold the numbers that are divisible by 3 or 5. Then we use a for loop starting at 1 and ending at number n and introduce an if statement that if x is divisible by 3 or 5, add x to the array. We iterate through the array until we have all the numbers between 1 and n. The full code for this is
function multiples(n){
var arr = []
for(var x = 1; x <= 20; x++){
if(x % 3 === 0 || x % 5 === 0){
arr.push(x)
}
}
return arr
}.
The next step is then call the array from the multiples function and add everything, and the function to do this is
function addition(arr){
var sum = 0
for(var x = 0; x < arr.length; x++){
sum += arr[x]
}
return sum
}.
With these two functions, we can return the sum of all multiples of 3 or 5. So let’s test it out. Let’s see if the code produces the correct array as above
[3, 5, 6, 9, 10, 12, 15, 18, 20].
From figure 1, we see it is indeed true

The sum of these numbers is 98, and figure 2 confirms that.

Now that we are done with the JavaScript part, we can move on to Ruby. With Ruby, it uses fewer lines so the solution is simply

You can see that in line 2, I added limit + 1 because I want to add 20 as well. On the right, we see the answer is 98, matching with the JavaScript solution and the top.
There is one more way to solve this, and that is using object oriented programming or OOP. In Ruby, we define a class we will call “Multiples”.
class Multiplesend.
We then initialize two variables limit and multiples and we set them to be equal to
def initialize(limit)
@limit = limit
@multiples = collect_multiples
end
and to make sure that we can call these variables, we need
attr_reader :limit, :multiples.
This will be added to the top of the class. The next two functions are similar to figure 2 except we do not need to call the limit because it is already called in the initialize function. It is simply
def collect_multiples
(1..limit).to_a.reject {|num| num unless num % 3 == 0 || num % 5 == 0}
end
def sum_multiples
multiples.inject(:+)
end
The full class is
class Multiples
attr_reader :limit, :multiples def initialize(limit)
@limit = limit
@multiples = collect_multiples
end def collect_multiples
(1..limit).to_a.reject {|num| num unless num % 3 == 0 || num % 5 == 0}
end
def sum_multiples
multiples.inject(:+)
end
end.
Now, let’s test this. We set limit to be 20, and the answer is indeed 98. See figure 4.
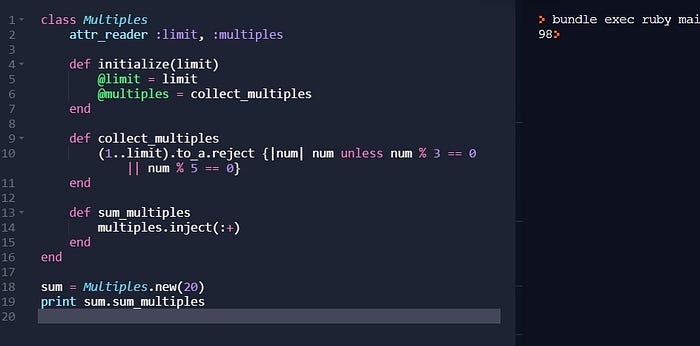
Now we have seen how to solve this algorithm using JavaScript, then Ruby then with OOP. Although the OOP route uses more lines, we don’t need to worry about calling the limit because it is called in the initialize function.